Filter fundamentals
Last updated onThis feature and document is applies to the server-side only. If you want to use client-side filter, see Client Filter.
MagicOnion provides a powerful feature called filters that allows you to hook into the service method invocation before and after. Filters provide a similar functionality to gRPC server interceptors, but with a more familiar programming model like HttpClient handlers or ASP.NET Core middlewares.
The following diagram illustrates the configuration of multiple filters being set and processed.
Implementation and Usage
To implement a filter, inherit from MagicOnionFilterAttribute
and implement the Invoke
method.
public class SampleFilterAttribute : MagicOnionFilterAttribute
{
public override async ValueTask Invoke(ServiceContext context, Func<ServiceContext, ValueTask> next)
{
try
{
/* on before */
await next(context); // next
/* on after */
}
catch
{
/* on exception */
throw;
}
finally
{
/* on finally */
}
}
}
In a filter, you call the next
delegate to call the next filter or the actual method. You can skip calling next
or catch exceptions from calling next
to add exception handling.
To apply the implemented filter, add an attribute to the class or method of the Unary service like [SampleFilter]
. You can also apply it globally to the entire application.
MagicOnion also provides an API for filters that is very similar to filters in ASP.NET Core MVC. These APIs support flexible filter implementations. For more information, see Filter Extensibility.
Global Filters
You can apply filters to the entire application by adding them to GlobalFilters
in MagicOnionOptions
.
services.AddMagicOnion(options =>
{
options.GlobalFilters.Add<MyServiceFilter>();
options.GlobalStreamingHubFilters.Add<MyHubFilter>();
});
Processing Order
Filters can be ordered and they are executed in the following order:
[Ordered filters] -> [Global filters] -> [Class filters] -> [Method filters]
Not ordered filters are treated as last (int.MaxValue
) and executed in the order in which they are added.
Integration with ASP.NET Core Middleware
In a MagicOnion server, you can also use ASP.NET Core middlewares. Middlewares are executed before filters because gRPC calls are processed as pure HTTP requests, while filters are executed after gRPC calls have started processing.
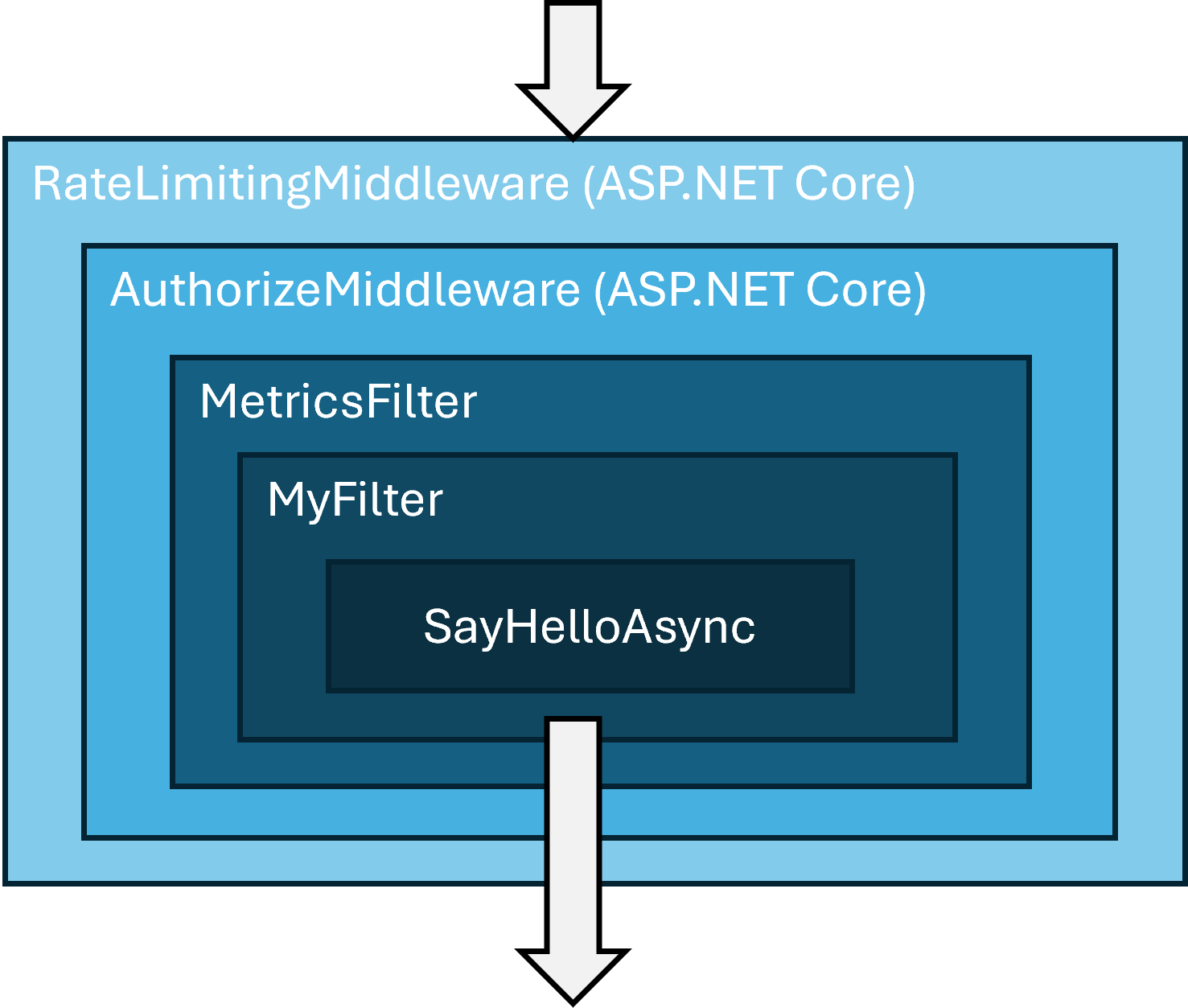